spring-resource源码剖析
Spring Resource
Why not Java URL类
原因: 对底层资源的支持不足。
there is no standardized URL implementation that may be used to access a resource that needs to be obtained from the classpath,or relative to a ServletContext.
不自定义URL handler的原因:
a. 过于复杂
b. lack some desirable functionality(如对URL所指资源是否存在的判断)
Resource 接口
1 |
|
继承体系
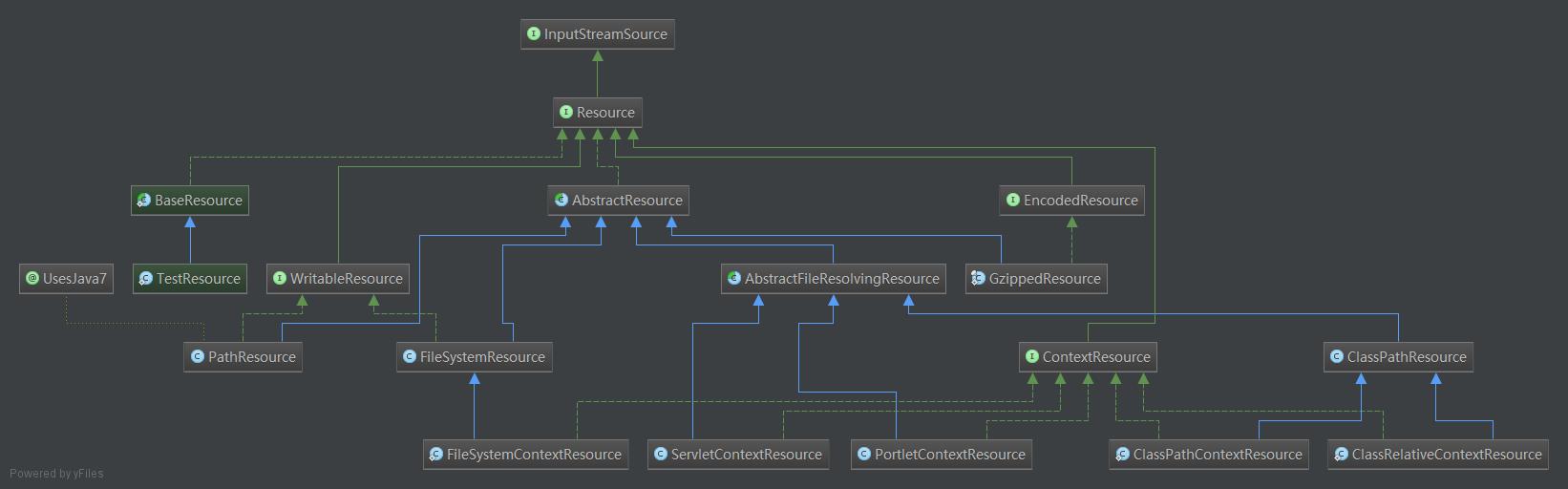
ResourceLoader
1 | public interface ResourceLoader { |
ResourceLoader 负责加载Resource, 所有的application context都实现了这个接口。
1 | Resource template = ctx.getResource("some/resource/path/myTemplate.txt"); |
如果上述的ctx的类型是 ClassPathXmlApplicationContext,那么返回的Resource的具体类型就是
ClassPathResource; 如果ctx的类型是FileSystemXmlApplicationContext, 返回的类型就变成了
FileSystemResource。
指定返回的Resource类型
1 | Resource template = ctx.getResource("classpath:some/resource/path/myTemplate.txt"); |
通过显式的指定classpath前缀,返回的Resource的实际类型就是 ClassPathResource
对应的关系见表格:
Prefix | Example | Explanation |
---|---|---|
classpath: | classpath:com/myapp/config.xml | Loaded from the classpath |
file: | file:///data/config.xml | Loaded as a URL, from the system |
http: | http://myserver/logo.png | Loaded as a URL |
(none) | /data/config.xml | Depends on the underlying ApplicationContext |
classpath*
classpath*:conf/appContext.xml
这个特殊的前缀会使spring在所有的ClassPath中查找和指定的名字相同的资源,他们会合并形成最终的
上下文。
This special prefix specifies that all classpath resources that match the given name must be obtained
(internally, this essentially happens via a ClassLoader.getResources(…) call), and then merged
to form the final application context definition.
ResourceLoaderAware
1 | public interface ResourceLoaderAware extends Aware { |
实现这个接口的类,可以获得所在容器的ResourceLoader实例,一般来说就是相应的Application Context。也可以当做
ApplicationContextAware的替代。
Interface to be implemented by any object that wishes to be notified of
the ResourceLoader (typically the ApplicationContext) that it runs in.
This is an alternative to a full ApplicationContext dependency via the
ApplicationContextAware interface.
除了实现上述接口,还可以使用基于类型的注入,将ResourceLoader注入到需要的地方。